Essential Software Architecture Patterns for Modern Applications
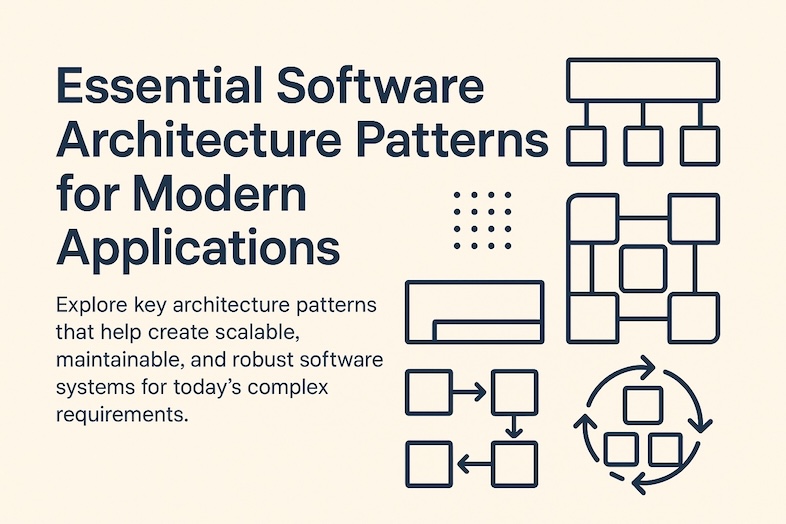
Essential Software Architecture Patterns for Modern Applications
Choosing the right architectural pattern is one of the most critical decisions in software development. The architecture you select influences everything from development speed to scalability, maintainability, and performance. In this article, I’ll explore several architectural patterns that have proven effective for modern applications.
Why Architecture Patterns Matter
Architecture patterns provide proven solutions to common design problems. They offer several benefits:
- Shared vocabulary - Teams can communicate more effectively about design
- Proven solutions - These patterns have been tested in real-world scenarios
- Guidance for decisions - They provide frameworks for making complex trade-offs
- Faster development - Teams don’t need to reinvent the wheel
Let’s examine some of the most valuable patterns for today’s software landscape.
Microservices Architecture
Microservices architecture has become the dominant pattern for large-scale applications, breaking down complex systems into smaller, independently deployable services.
┌────────────────┐ ┌────────────────┐ ┌────────────────┐
│ User Service │ │ Order Service │ │Product Service │
│ │ │ │ │ │
│ Database │ │ Database │ │ Database │
└────────────────┘ └────────────────┘ └────────────────┘
│ │ │
└──────────┬───────┴─────────┬────────┘
│ │
┌──────────▼─────────┐ ┌▼────────────────┐
│ API Gateway │ │ Event Bus │
└────────────────────┘ └─────────────────┘
Key characteristics:
- Service independence - Each service can be developed, deployed, and scaled independently
- Domain-focused - Services are organized around business capabilities
- Decentralized data - Each service typically manages its own database
- API-based communication - Services interact through well-defined APIs
When to use:
- Large, complex applications with distinct functional domains
- Teams distributed across different locations or organizational units
- Applications requiring different scaling needs for different components
- Systems needing a high degree of resilience and fault isolation
Implementation considerations:
// Example of service communication in a microservices architecture
// User Service API endpoint
app.get("/api/users/:id", async (req, res) => {
try {
const user = await getUserById(req.params.id);
// Call the Order Service to get user's orders
const ordersResponse = await fetch(
`${ORDER_SERVICE_URL}/api/orders?userId=${user.id}`,
{
headers: {
Authorization: `Bearer ${generateServiceToken()}`,
"X-Request-ID": req.headers["x-request-id"] || uuidv4()
}
}
);
if (!ordersResponse.ok) {
// Handle service unavailability gracefully
return res.json({
...user,
orders: { error: "Orders temporarily unavailable" }
});
}
const orders = await ordersResponse.json();
res.json({
...user,
orders
});
} catch (error) {
console.error("Error fetching user data:", error);
res.status(500).json({ error: "Internal server error" });
}
});
Event-Driven Architecture
Event-driven architecture focuses on the production, detection, and reaction to events that represent significant changes in state.
┌────────────┐ ┌─────────────┐ ┌────────────┐
│ Producer │────▶│ Event Broker │────▶│ Consumer A │
└────────────┘ │ │ └────────────┘
│ │ ┌────────────┐
│ │────▶│ Consumer B │
└─────────────┘ └────────────┘
Key characteristics:
- Loose coupling - Producers don’t know which consumers will process their events
- Asynchronous processing - Events are processed independently of their creation
- Scalable - Easy to add new producers or consumers without system-wide changes
- Reactive - The system responds to events as they occur
When to use:
- Real-time data processing requirements
- Highly scalable systems with unpredictable load
- Complex systems with many interconnected components
- Applications where components should be decoupled
Implementation considerations:
// Example using a message broker like Kafka or RabbitMQ
// Producer code
async function createOrder(orderData) {
// Save order to database
const order = await orderRepository.save(orderData);
// Publish event
await eventBus.publish("order-created", {
orderId: order.id,
userId: order.userId,
totalAmount: order.totalAmount,
timestamp: new Date().toISOString()
});
return order;
}
// Consumer code
eventBus.subscribe("order-created", async (event) => {
// Process the event
await notificationService.sendOrderConfirmation(
event.userId,
event.orderId,
event.totalAmount
);
// Update analytics
await analyticsService.trackOrder(event);
});
Hexagonal Architecture (Ports and Adapters)
Hexagonal architecture, also known as Ports and Adapters, separates the core application logic from external concerns like databases, UIs, and third-party services.
┌───────────────────────────┐
│ │
┌─────────▶│ Application Core │◀────────┐
│ │ │ │
│ └───────────────────────────┘ │
│ ▲ │
│ │ │
│ ▼ │
│ ┌───────────────────────────┐ │
│ │ Ports │ │
│ └───────────────────────────┘ │
│ ▲ │
│ │ │
▼ ▼ ▼
┌─────────┐ ┌─────────┐ ┌─────────┐ ┌──────────┐
│ UI │ │ Database│ │ API │ │ External │
│ Adapter │ │ Adapter │ │ Adapter │ │ Services │
└─────────┘ └─────────┘ └─────────┘ └──────────┘
Key characteristics:
- Domain-centric - The core application logic is isolated from external concerns
- Dependency inversion - The core defines interfaces (ports) that adapters implement
- Testability - The core business logic can be tested without external dependencies
- Flexibility - External components can be replaced without affecting the core
When to use:
- Complex business logic that should be isolated from technical details
- Applications that need to support multiple UIs, databases, or external services
- Systems where testability is a primary concern
- Projects that need to evolve over time with changing requirements
Implementation considerations:
// Core domain model and business logic
interface UserRepository {
findById(id: string): Promise<User | null>;
save(user: User): Promise<User>;
}
class UserService {
constructor(private userRepository: UserRepository) {}
async changeEmail(userId: string, newEmail: string): Promise<User> {
const user = await this.userRepository.findById(userId);
if (!user) {
throw new Error("User not found");
}
if (!this.isValidEmail(newEmail)) {
throw new Error("Invalid email format");
}
user.email = newEmail;
user.updatedAt = new Date();
return this.userRepository.save(user);
}
private isValidEmail(email: string): boolean {
// Email validation logic
return /^[^\s@]+@[^\s@]+\.[^\s@]+$/.test(email);
}
}
// Adapter implementation for MongoDB
class MongoUserRepository implements UserRepository {
constructor(private db: mongodb.Db) {}
async findById(id: string): Promise<User | null> {
const result = await this.db
.collection("users")
.findOne({ _id: new ObjectId(id) });
return result ? this.mapToUser(result) : null;
}
async save(user: User): Promise<User> {
// Implementation details...
}
private mapToUser(document: any): User {
// Mapping logic...
}
}
Backend for Frontend (BFF)
The Backend for Frontend pattern creates specialized backend services tailored to specific frontend needs, typically organized by client type.
┌───────────┐ ┌───────────┐ ┌───────────┐
│ Mobile │ │ Web App │ │ Desktop │
│ Client │ │ Client │ │ Client │
└─────┬─────┘ └─────┬─────┘ └─────┬─────┘
│ │ │
┌─────▼─────┐ ┌─────▼─────┐ ┌─────▼─────┐
│ Mobile │ │ Web │ │ Desktop │
│ BFF │ │ BFF │ │ BFF │
└─────┬─────┘ └─────┬─────┘ └─────┬─────┘
│ │ │
└────────┬────────┴─────────┬──────┘
│ │
┌──────▼──────┐ ┌──────▼──────┐
│ Microservice│ │ Microservice│
│ A │ │ B │
└─────────────┘ └─────────────┘
Key characteristics:
- Client-specific - Each backend service is tailored to a specific frontend
- Optimized queries - BFFs aggregate data from multiple services in the most efficient way for each client
- Simplified clients - The complexity of service orchestration is moved from client to server
- Independent evolution - Each BFF can evolve alongside its client without affecting others
When to use:
- Applications with multiple client types (web, mobile, desktop)
- Clients with significantly different data needs
- Microservice architectures where clients need data from multiple services
- Systems where client performance is critical
Implementation considerations:
// Mobile BFF - Optimized for mobile clients
app.get("/api/mobile/user-dashboard", async (req, res) => {
try {
// Get user data with minimal fields needed for mobile
const user = await userService.getBasicProfile(req.userId);
// Get recent activity - limit to 5 for mobile
const recentActivity = await activityService.getRecent(req.userId, 5);
// Only include high-priority notifications for mobile
const notifications = await notificationService.getHighPriority(req.userId);
// Combine data into a single response optimized for mobile
res.json({
user: {
name: user.name,
profileImage: user.profileImageSmall
},
recentActivity,
notificationCount: notifications.length
});
} catch (error) {
res.status(500).json({ error: "Internal server error" });
}
});
// Web BFF - More comprehensive data for larger screens
app.get("/api/web/user-dashboard", async (req, res) => {
try {
// Get full user profile for web
const user = await userService.getFullProfile(req.userId);
// Get more activity items for web display
const recentActivity = await activityService.getRecent(req.userId, 20);
// Include all notifications for web
const notifications = await notificationService.getAll(req.userId);
// Include analytics data only needed for web dashboard
const analytics = await analyticsService.getUserStats(req.userId);
// Return comprehensive data for web interface
res.json({
user,
recentActivity,
notifications,
analytics
});
} catch (error) {
res.status(500).json({ error: "Internal server error" });
}
});
CQRS (Command Query Responsibility Segregation)
CQRS separates read operations (queries) from write operations (commands), allowing each to be optimized independently.
┌─────────┐ ┌──────────────┐
│ Command │────────▶│ Command │
│ API │ │ Handlers │
└─────────┘ └──────┬───────┘
│
▼
┌──────────────┐
│ Write Model │
└──────┬───────┘
│
▼
┌──────────────┐
│ Events │
└──────┬───────┘
│
▼
┌─────────┐ ┌──────────────┐
│ Query │◀────────│ Read Model │
│ API │ │ │
└─────────┘ └──────────────┘
Key characteristics:
- Separate models - Different data models for reading and writing
- Optimized performance - Read models can be denormalized for query performance
- Scalability - Read and write workloads can be scaled independently
- Complexity - Adds complexity due to maintaining multiple models
When to use:
- Applications with significant imbalance between read and write operations
- Systems requiring high performance for queries
- Complex domains where the read model and write model have different structures
- Applications using event sourcing
Implementation considerations:
// Command handler (write side)
class CreateOrderCommandHandler {
constructor(
private orderRepository: OrderRepository,
private eventBus: EventBus
) {}
async handle(command: CreateOrderCommand): Promise<string> {
// Validate command data
this.validateCommand(command);
// Create new order entity
const order = new Order(
generateOrderId(),
command.userId,
command.items,
command.shippingAddress
);
// Apply business rules
order.calculateTotals();
// Save to write model
await this.orderRepository.save(order);
// Publish events for updating read models
await this.eventBus.publish(new OrderCreatedEvent(order));
// Return order ID
return order.id;
}
private validateCommand(command: CreateOrderCommand): void {
// Validation logic
}
}
// Query handler (read side)
class GetOrdersQueryHandler {
constructor(private orderReadModel: OrderReadModel) {}
async handle(query: GetOrdersQuery): Promise<OrderSummary[]> {
// Get data from optimized read model
return this.orderReadModel.findByUserId(
query.userId,
query.page,
query.pageSize,
query.sortBy
);
}
}
// Event handler to update read model
class OrderReadModelUpdater {
constructor(private orderReadModel: OrderReadModel) {}
async handleOrderCreated(event: OrderCreatedEvent): Promise<void> {
// Create denormalized order summary for read model
await this.orderReadModel.insert({
id: event.orderId,
userId: event.userId,
date: event.createdAt,
status: "pending",
totalAmount: event.totalAmount,
itemCount: event.items.length
// Other denormalized data needed for efficient querying
});
}
}
Conclusion
Each of these architecture patterns has its strengths and appropriate use cases. The most successful applications often combine elements from multiple patterns to address specific requirements.
When choosing an architecture pattern, consider:
- Current and future scale - Some patterns only make sense at certain scales
- Team structure and expertise - Choose patterns that align with your team’s knowledge
- Business requirements - Different domains have different architectural needs
- Performance characteristics - Consider the performance profile your application needs
- Development velocity - Some patterns require more upfront design but improve long-term velocity
Remember that architecture is not a one-time decision but an ongoing process of refinement. Start with a pattern that addresses your core requirements, but be prepared to evolve as your understanding of the problem domain deepens.
What architecture patterns have you found most valuable in your projects? Share your experiences in the comments below!